This program show you how to convert temperatures in Python. It uses a menu system to allow you to select an option. Or, you can also end the program. In addition, you get to see the match..case statement in action.
Code listing to convert temperatures in Python
Below is the code listing for this program.
# ---------------------------------------------------------------------
# convert_temps.py
# Designed and programmed by Alex Shaw III
# Date created: January 5, 2023
# Last modified: January 5, 2023
#
# This program allows you to convert Celsius, Fahrenheit, and Kelvin
# temperatures from a menu
# ---------------------------------------------------------------------
# -----------------
# Define menu items
# -----------------
menuItems = ['A. Celsius to Fahrenheit', 'B. Fahrenheit to Celsius',
'C. Celsius to Kelvin', 'D. Exit program']
# ----------------
# Define functions
# ----------------
def mainMenu():
print('\nMain menu\n' + '-'*40)
for menuItem in menuItems:
print(menuItem)
print('-'*40, '\n')
# ------------
# Main program
# ------------
end = False
while (end == False):
mainMenu()
choice = input('Please make a selection: ')
match choice.upper():
case 'A':
print('\n****** Convert Celsius to Fahrenheit ******\n')
celTemp = input('Enter a Celsius temperature: ')
fahTemp = (float(celTemp) * (9 / 5)) + 32
print(str(celTemp) + ' degrees Celsius is ' +
str(fahTemp) + ' degrees Fahrenheit.\n')
case 'B':
print('\n****** Convert Fahrenheit to Celsius ******\n')
fahTemp = input('Enter a Fahrenheit temperature: ')
celTemp = (float(fahTemp) - 32) * (5 / 9)
print(str(fahTemp) + ' degrees Fahrenheit is ' +
str(celTemp) + ' degrees Celsius.\n')
case 'C':
print('\n****** Convert Celsius to Kelvin ******\n')
celTemp = input('Enter a Celsius temperature: ')
kelTemp = float(celTemp) + 273.15
print(str(celTemp) + ' degrees Celsius is ' +
str(kelTemp) + ' Kelvin.\n')
case 'D':
print('\n****** End of program ******')
print('Thank you for using this program.\n')
end = True
case _:
print('\n****** Invalid selection ******')
print('Please try again.\n')
Output
Here is the output for the above program.

Developer’s note
Instead of putting all that code in the case routines, you can also create functions for each case routine.
If you have any questions or comments, then send us an email at support@techronology.com.
Related
- Convert Celsius to Fahrenheit in Python
- Convert Celsius to Kelvin in Python
- Convert Fahrenheit to Celsius in Python
Python page
Click on the button below to go to our Python page.
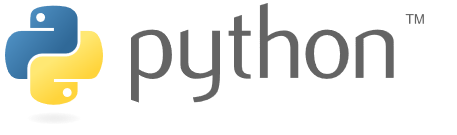
Python is a high-level computer programming language. If you want more information on Python, then visit Python Software Foundation.