The code listing in this reference shows you how to create a menu in Python.
Although we consider this a basic program, you may find some advanced techniques presented in the code. Particularly, on the last line.
Code listing to create a menu in Python
Below is the code listing for this program.
# -----------------------------------------------------------------------------
# menu.py
# Designed and programmed by Alex Shaw III
# Date created: January 4, 2023
# Last modified: January 4, 2023
#
# This program creates a basic menu to select options by entering a character
# -----------------------------------------------------------------------------
# ------ Variables ------
# Define menu items
# Depending on the length, you can probably put these on one line
menuItems = ['A. Menu item 1',
'B. Menu item 2',
'C. Menu item 3']
# ------ Menu ------
print('Main menu\n' + '-'*40) # print menu title and top border
for menuItem in menuItems: # cycle through menu items
print(menuItem) # show menu item
print('-'*40) # create bottom border
choice = input('Please enter your choice: ') # get user selection
# ------ Show selection ------
# Of course, this section is mainly for testing purposes
print('\nYou selected menu item ' + choice.upper() + ':') # show uppercase choice character
print(menuItems[('A', 'B', 'C').index(choice.upper())][4:]) # show menu item value of choice
Output
Here is the output for the above program.

Developer’s note
Notice the technique we used on the last line, to extract the text from the selected menu item. Of course, you may have to break it out in pieces to see what is going on.
If you have any questions or comments, then send us an email at support@techronology.com.
Python page
Click on the button below to go to our Python page.
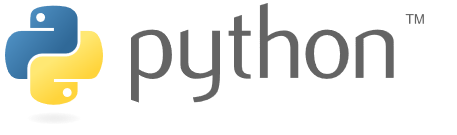
Python is a high-level computer programming language. If you want more information on Python, then visit Python Software Foundation.
Related
- Convert Celsius to Fahrenheit in Python
- Create a Pac-Man figure in Python
- Create a window in Python
- Percent of change in Python
- Solve basic linear equation Ax+B=C in Python